Smart Connectors for the Next Generation Internet
What are the benefits of using Reowolf connectors over BSD-style sockets for Internet applications?
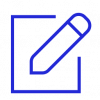
Declarative Protocol Language
Applications declare the network's behavior it expects from the connector declaratively and ahead-of-time, in a domain specific language:
Protocol Description Language (PDL).
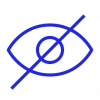
Privacy-first Quality of Service
A Reowolf implementation offers optimization opportunities and intrusion detection capability that does not rely on ad-hoc and non-standard techniques such as Deep Packet Inspection (DPI).
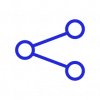
Decentralization
Programming distributed Internet applications using connectors is simpler than using sockets: applications use only a single connector to manage communication with multiple peers.
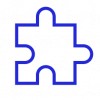
Compositionality
Connectors are compositionally built from user-definable protocol components, that improves reusability and allows for formal verification of high-level protocol properties.
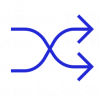
Compatibility & Interoperability
Connectors can run seamlessly on existing Internet infrastructure, and is interoperable with socket-based applications using current and novel transport layer implementations.
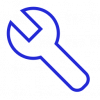
Operating System Integration
Reowolf aims at building two standardized implementations, following the development model of pthreads: a user-mode reference implementation and a kernel-mode driver.
Mailing List
A public mailing list is available for general discussion:
You can use your own e-mail client to send a message, or the contact form. The mailing list is moderated: your message first gets queued and approved.
Subscription to the mailing list requires confirmation of your e-mail address.
All approved messages are stored in the public list archive.
Contact form
The message you send via this form will be posted to the public mailing list. This mailing list is moderated, so it may take some time before your message is received and replied to.
Reowolf 1.0 has received funding from NGI ZERO PET Fund, a fund established by NLnet Foundation with financial support from the European Commission, as part of the Horizon 2020 Research and Innovation Programme, under grant agreement No. 825310.
Reowolf 2.0 has received funding from NGI POINTER, the Next Generation Internet Program for Open Internet Renovation, with financial support from the European Commission, as part of the Horizon 2020 Research and Innovation Programme, under Grant Agreement No. 871528.
Verified Reowolf has received funding from NGI Assure Fund, a fund established by NLnet Foundation with financial support from the European Commission’s Next Generation Internet programme, under the aegis of DG Communications Networks, Content and Technology under grant agreement No. 957073.